Python’s range function returns a series of values that begin at the input value (default: 0), increase at the designated step size (default: 1), and end before the input value. This post will go over range functions in Python and how to use them.
The range() function in Python is a fundamental tool used for generating a sequence of numbers, especially within loops like for in range or in range Python statements. Whether you’re writing basic iterations or advanced logic, understanding what is range in Python and its various forms—like python for range, python inrange, or even ranges in Python—is crucial for writing clean, efficient code. This guide breaks it down simply with examples. Want to become a Python expert? Join our hands-on Python Training in Pune at 3RI Technologies and turn coding into your career.
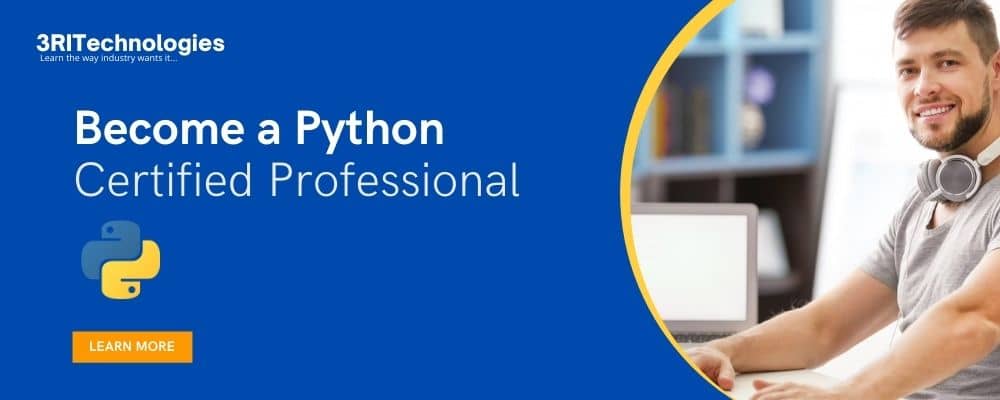
How to Use range() Function in Python Effectively
1.Fundamentals of Python Range
The Python function range() is a frequently used and well-liked tool, especially when working with for and while loops. It returns a series of numbers and is immutable, meaning that its value cannot be changed.
2. Python range Syntax
range(start, stop, step)
3. Python range() function
The range() method in Python yields a series of values inside a specified range.It is most typically used with Python loops to repeat sequences over a sequence of numbers.Check out the Python Training in Pune..
4. Parameters of Range Python
Because the range() function simply keeps the start, stop, and step values, it uses less memory than tuples or lists. The range() function requires three range parameters.
range(stop_value) | This defines the range() function, which returns a sequence of numbers and terminates at the specified stop_value. By default, it considers the starting value to be 0. |
range(start_value, stop_value) | This definition of the range() function yields a sequence of numbers that begins at the supplied start value, increases by 1, and ends at the supplied stop_value. |
range(start_value, stop_value, step_value) | This definition of the range() function yields a series of numbers from the supplied start value to the stop value; the step value is equal to the difference between the numbers. |
5. Syntax of Python range() function
Syntax: range(start, stop, step)
Parameter :
- start: [optional] The sequence’s start value
- stop: the value that follows the sequence’s final value
- step: [optional] integer value that represents the variation between any two numbers in the series
Return: Provides an object representing a series of numbers in return.
5. Why is the range function in Python used?
When it comes to building mathematical sequences, Python’s range function is a very useful tool.
It is sometimes used in loops, for example, to loop over these chains and do particular tasks. By selecting the start, stop, and step values, you can quickly generate a range of whole integers that satisfy your particular needs.
The most common usage of Python’s range() function is to create circling lists. You can easily access the components of a succession by file while repeating them using the range() method with the len() function.
Looping indexes are another application for the range function. By combining the range function with a loop, you may retrieve a sequence’s elements quickly.
The range function could come in handy when you wish to make a series of requests with increasing numbers. By giving the step value, you can alter the augmentation or decrease between each number in the series.Explore further with our Python Web Development Course to deepen your knowledge and skills.
6. Python range (stop)
Python’s range(stop) function provides a range of numbers from 0 to the specified stop value, but not higher. This proves that the range can recall any integer between zero and stop-1.
The following is an illustration of the range(stop) capability capabilities:
for I in range(5):
print(i)
Output:
0
1
2
3
4
In the example above, the range(5) function progresses entire numbers from 0 to 4. The ‘for’ loop then highlights this list by assigning the variable “I” to each entire number. After each iteration of the loop, the print command outputs the value of “I.”
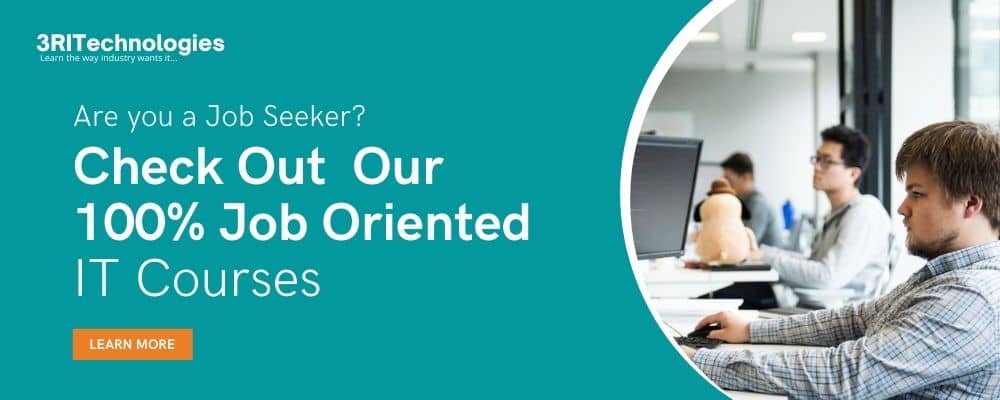
7. Python range (start, stop)
With the Python range(start, stop) approach, you may create a sequence of whole numbers starting from the given start value and ending with the predefined stop value. For any integer between start and stop-1, the range will remember it.
This shows the (start to stop) range of function:
for I in range(2, 8):
print(i)
Output:
2
3
4
5
6
7
The range (2, 8) function in this paradigm advances the integers from 2 to 7. The ‘for’ loop then starts iterating through this list, assigning each integer to the variable “I” at that point. Every iteration of the loop produces the value “I,” according to the print statement.
8. Python range (start, stop, step)
You can use Python’s range(start, stop, step) method to produce a sequence of numbers that starts with the specified start value and ends with the predefined completion value. The border between steps determines the addition factor for each integer in the series.
This is an example of how range(start, stop, step) feature works:
for I in range(1, 10, 2):
print(i)
Output
1
3
5
7
9
In this instance, the range(1, 10, 2) function produces a series of values that start at 1 and go up by 2 until they are less than 10. As a result, the numbers are 1, 3, 5, 7, and 9. The for loop then uses the print command to output each integer one at a time on a separate line.Looking forward to becoming a Python Developer? Then get certified with Python Online Training
9. Incrementing the Range Using A Positive Step
One must have steps that are a positive number in order to increase.
# incremented by 4
for i in range(0, 30, 4):
print(i, end=” “)
print()
Output :
0 4 8 12 16 20 24 28
10. Python range() with Float Values
The range() method in Python does not accept floating-point numbers. i.e., the user’s parameters cannot contain floating-point or non-integer numbers. A user can only enter whole numbers.
# using a float number
for i in range(3.3):
print(i)
Output :
for i in range(3.3):
TypeError: ‘ It is impossible to interpret a float object as an integer .Learn more at Full Stack Online Course
11. Python range() with More Examples
● Concatenation two range() methods with the itertools chain() method
You can use the itertools module’s chain() method to concatenate the results of two range() methods. Using its arguments, the chain() method prints each value in the iterable targets one after the other.
from itertools import chain
# Using chain method
print(“Concatenating the result”)
res = chain(range(5), range(10, 20, 2))
for i in res:
print(i, end=” “)
Output :
Concatenating the result
0 1 2 3 4 10 12 14 16 18
● Accessing range() using an index value
The range() function returns a list of numbers as its object, which is accessible by its index value. Its target is compatible with both negative and positive indexing.
ele = range(10)[0]
print(“First element:”, ele)
ele = range(10)[-1]
print(“\nLast element:”, ele)
ele = range(10)[4]
print(“\nFifth element:”, ele)
Output :
First element: 0
Last element: 9
Fifth element: 4
● Use the range() function with a List in Python
Here, we show you how to make a list and print its elements using Python’s range() function.
fruits = [“apple”, “banana”, “cherry”, “date”]
for i in range(len(fruits)):
print(fruits[i])
Output :
apple
banana
cherry
date
Enroll in the Data Analyst course in Pune to master Data Analytics.
12. Important Reminders Regarding the range() Function in Python
The flexible Python range() method may help you create a numerical sequence based on the start, stop, and step values you provide. You should take into consideration the following important range() function guidelines:
- A sequence of numbers is provided by the range() function, beginning at the beginning value and ending at the stop value.
- Naturally, the step value starts at 1. This illustrates that the grouping will increase by 1 with each repetition.
- You can use any number of floating-point numbers for the start, stop, and step values.
- The range() function returns an iterable item; you may transform it into a list or any other type of data using the list() function.
- To iterate through a list of numbers, loops often employ the range() method.
- Using the list value as information enclosed in square sections [] after the range() function, you can access particular elements inside a range. To master the skills from industry experts at 3RI Technologies.
The Bottom Line
In conclusion, organizing whole numbers is a strong suit for the Python range() function. Characterizing the beginning and end values allows for the quick creation of a progression of whole numbers within a specified range. Whether you actually want to generate a sequence of integers for various uses or simply want to repeat something over and over, the range() function is a vital tool to have in your toolbox of programming tools.